This took me a LOT longer then it should have to figure out. So for anyone else wanting to run the 64bit versions of Windows on Qemu (I havent tested Vista/2008/7 yet) Only version 0.9.0 will work.
Because sourceforge is still giving me errors I’ll provide direct links…
Anyways to buidl Qemu you’ll need a MinGW/MSYS enviroment. The new stuff works on Vista x64 so that’s good to me, as it’ll run natively.
You’ll need the following files:
MinGW-5.1.4.exe
MSYS-1.0.11-rc-1.exe
msysDTK-1.0.1.exe
w32api-3.13-mingw32-dev.tar.gz
mingwrt-3.15.2-mingw32-dev.tar.gz
First, install MinGW by choosing the ‘current’ version, then check the following options:
*MinGW Base tools
*G++ compiler
*MinGW make
Allow it to instal into c:\MinGW
Next install MSYS with the default options. Then it’ll ask you the following, respond as I have:
Do you wish to continue with the post install? [yn ] y
Do you have MinGW installed? [yn ] y
Please answer the following in the form of c:/foo/bar.
Where is your MinGW installation? c:/mingw
Install msysDTK with the default options.
Now you should be able to run the msys CLI
Start -> run -> mingw -> msys -> msys
Let’s expand out the win32api & mingw32 dev updates:
cd /mingw
tar -zxvf /c/install/qemu-build/w32api-3.13-mingw32-dev.tar.gz
tar -zxvf /c/install/qemu-build/mingwrt-3.15.2-mingw32-dev.tar.gz
Now your ‘gcc -v’ should return something like this:
Reading specs from c:/mingw/bin/../lib/gcc/mingw32/3.4.5/specs
Configured with: ../gcc-3.4.5-20060117-3/configure –with-gcc –with-gnu-ld –wi
th-gnu-as –host=mingw32 –target=mingw32 –prefix=/mingw –enable-threads –dis
able-nls –enable-languages=c,c++,f77,ada,objc,java –disable-win32-registry –d
isable-shared –enable-sjlj-exceptions –enable-libgcj –disable-java-awt –with
out-x –enable-java-gc=boehm –disable-libgcj-debug –enable-interpreter –enabl
e-hash-synchronization –enable-libstdcxx-debug
Thread model: win32
gcc version 3.4.5 (mingw-vista special r3)
Ok, now let’s build the prerequisits, zlib & SDL.
zlib-1.2.3.tar.gz
cd /
mkdir -p /usr/src
cd /usr/src
tar -zxvf /c/install/qemu-build/zlib-1.2.3.tar.gz
./configure
make
make install
Now SDL.
SDL-1.2.13.tar.gz
tar -zxvf /c/install/qemu-build/SDL-1.2.13.tar.gz
cd SDL-1.2.13
./configure
make
make install
Now we need to tweak some things that MinGW seems to have issues finding in the /usr/local path.. I’m sure there is a better ‘fix’ but hell, this is quick & cheap!
cd /mingw/include
ln -s /usr/local/include/zconf.h .
ln -s /usr/local/include/zlib.h .
ln -s /usr/local/include/SDL .
cd /mingw/lib
ln -s /usr/local/lib/libSDL.a .
ln -s /usr/local/lib/libz.a .
cd /bin
ln -s true.exe texi2html.exe
ln -s true.exe pod2man.exe
Ok, now we just need the source to Qemu 0.9.0…. It’s becoming something RARE which is weird considering just how compatable this version is… So I’d recommend keeping a copy in email or something.
qemu-0.9.0.tar.gz
cd /usr/src
tar -zxvf /c/install/qemu-build/qemu-0.9.0.tar.gz
cd qemu-0.9.0
./configure –target-list=x86_64-softmmu
make
Now instead of the usual Qemu 32bit x86 emulator, you’ll get qemu-system-x86_64.exe in the x86_64-softmmu directory. Running it is just like the regular Qemu. So first I’m going to create a 16GB disk to boot from like this:
qemu-img create -f qcow win64.disk 16G
*NOTE if you have any issues where it just doesn’t work, use the qemu-img from here. I’ve had issues with the one that I’ve built, but the emulator works…. go figure.
Now let’s boot from the disc:
$ x86_64-softmmu/qemu-system-x86_64.exe -m 1024 -L pc-bios/ -hda win64.disk -cdrom en_win_srv_2003_r2_enterprise_x64_cd1.iso -net nic,model=rtl8139 -net user -boot d
Now if you don’t have the ISO files, and have physical discs don’t fret! It’s easy to have Qemu point to them… Let’s say your CD-ROM (DVD/BR disk) is D: then it’s just a matter of running:
$ x86_64-softmmu/qemu-system-x86_64.exe -m 1024 -L pc-bios/ -hda win64.disk -cdrom \\.\d: -net nic,model=rtl8139 -net user -boot d
Easy, right? Remember the -m flag for memory, otherwise your VM will run in a TINY 128mb of ram.. And it’ll be insanely SLOW.
And then you’ll get this!

The first screen.. It doesn’t sound all that 64 bit does it?

Now we are talking! It certainly is the 64 bit version… It reminds me of the PowerPC/MIPS/Alpha builds where once the Kernel has loaded, it’s all Windows NT..

Select your partition, and let’s format away!

Time for the file copy… This will take a while.
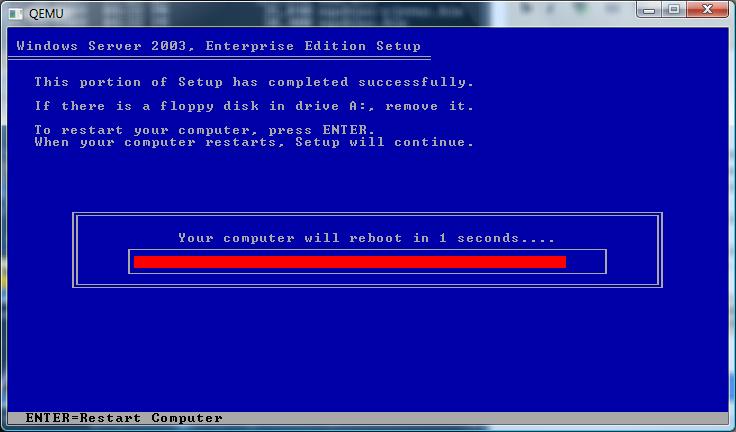
Finally!
Then it’ll reboot, and you’ll get the happy bootloader!
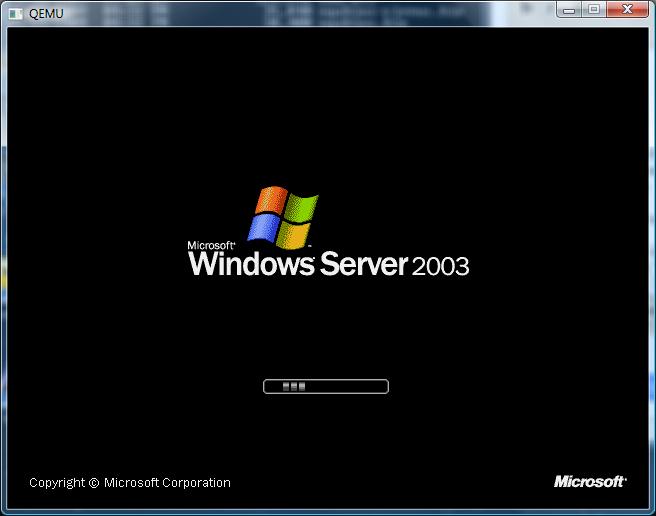
Bootloader in action..
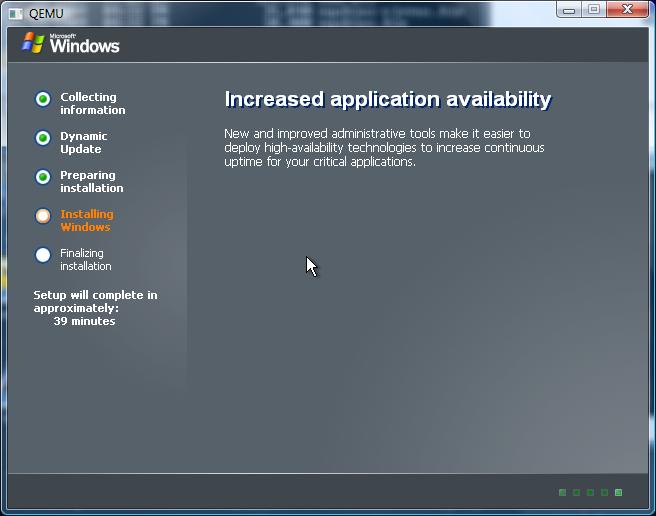
I haven’t timed it, but I suspect it’ll be longer then 39 minutes.
As you can see with the right version of Qemu it’s trivial to get Windows 2003 r2 x64 running… It’s good for doing some .net 32/64 bit testing… Which reminds me of another tidbit..
Some things in .net land will NOT work on IIS running in 64 bit mode. You’ll have to throw the switch to get a 32bit .net on IIS. The good news though is that this can take advantage of 2GB for a normal exe, and if you tag it, 3GB to under 4GB of ram.. So the 64bit version is not without waste.
%SystemRoot%\Microsoft.NET\Framework\v2.0.50727\aspnet_regiis -i -enable
iisreset
CScript “%SystemDrive%\InetPub\AdminScripts\adsutil.vbs” set w3svc/AppPools/Enable32bitAppOnWin64 1
iisreset
I did verify that this would get sharepoint to run on 2003 x64.. As I always feel better trashing a VM then real iron…
And don’t forget the flexibility of the -redir command on Qemu to allow you to redirect ports into the VM…
Say you want to use terminal server into your VM, you can redirect say port 1000 into the vm by adding:
-redir tcp:10000:10.0.2.15:3389
Then it’s a simple matter of using a terminal server client to localhost:10000
I hope this clears up how easy it is to build your own Qemu, and of course how to run something other then the ‘normal’ 32bit version of Qemu.