One of the things that always annoyed me about DooM is the fixed point math. It relies on a 64bit data type, which many 32bit platform just lack. Namely the FixedMul & FixedDiv.
fixed_t FixedMul
( fixed_t a,
fixed_t b )
{
return ((long long) a * (long long) b) >> FRACBITS;
}
They are generally re-written into assembly, at least for the i386 getting around the whole 64bit on a 32bit platform.
FixedMul:
pushl %ebp
movl %esp,%ebp
movl 8(%ebp),%eax
imull 12(%ebp)
shrdl $16,%edx,%eax
popl %ebp
ret
FixedDiv2:
pushl %ebp
movl %esp,%ebp
movl 8(%ebp),%eax
cdq
shldl $16,%eax,%edx
sall $16,%eax
idivl 12(%ebp)
popl %ebp
ret
So that’d always been the ‘catch’ when porting Doom is that you either need a ‘long long’ data type, or the custom assembly or you basically are out of luck.
I know I’m a weird person, but I do like going backwards in terms of software, while most people want latest GCC 14 targeting old machines or some other hack, I prefer the opposite, trying to get the oldest stuff running on something new(er). In this case, it’s GCC 1.27.
While much of the old GCC history is lost, I did my best to collect as many versions as I could find here, along with doing patches in reverse to ‘reconstruct’ many old versions. The results are on archive.org. And what is significant from this, is the first version of GCC with i386 support appears in version 1.27.
From the internals-1 file we can find out that we all have William Schelter to thank for doing the bulk of the 386 port of GCC.
William Schelter did most of the work on the Intel 80386 support.
Internals-1 – gcc 1.27
With the first commit being on May 29th, 1988:
Sun May 29 00:20:23 1988 Richard Stallman (rms at sugar-bombs.ai.mit.edu)
...
* tm-att386.h: New file.
GCC 1.25
The first GCC with i386 parts is 1.25, however it tends to emit the instruction movsbl, which GAS doesn’t like. Comparing the output to GCC 1.40 reveals this:
- movsbl -12(%ebp),%ax
+ movsbw -12(%ebp),%ax
It’s trivial enough to change to a movsbw, but there are some other issues going on, and even the Infocom ’87 interpreter won’t fully build & run. The files input.c & print.c have to be built with a later version of GCC, in this case I used GCC 1.40.
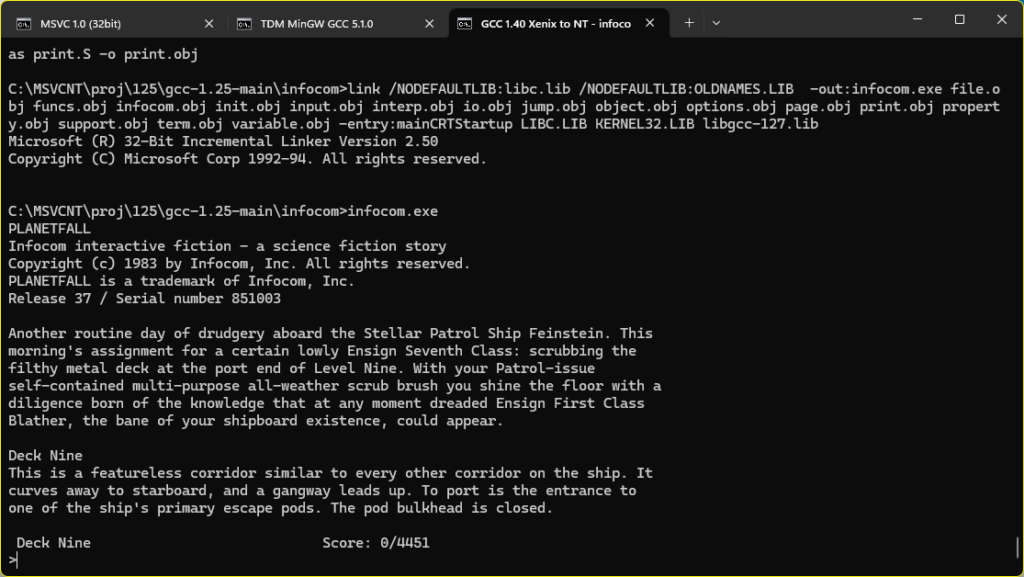
However when using the old XenixNT build thing I did, I do get a runnable EXE!
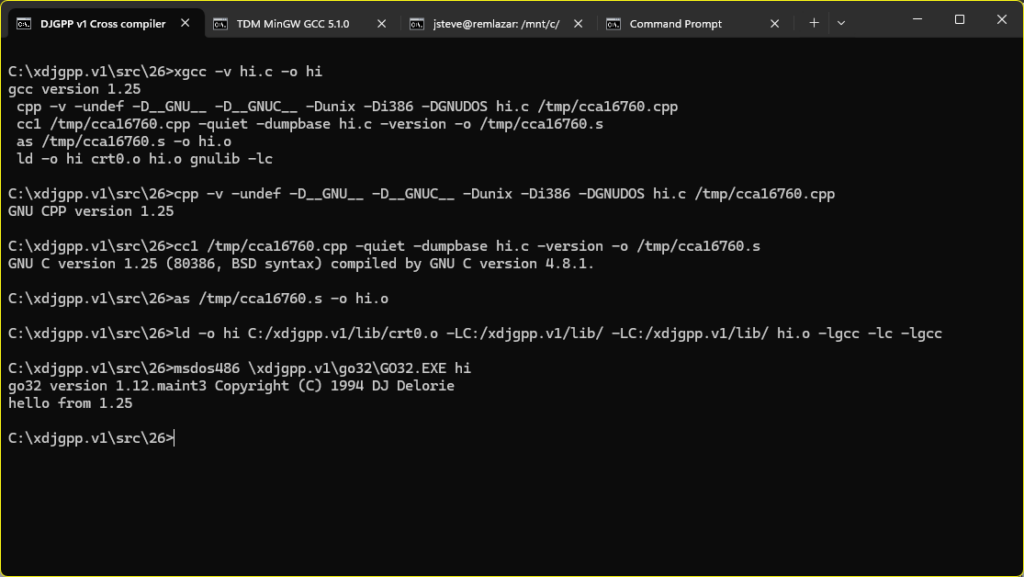
I added the BSD targeting files from 1.27 allowing it to generate the needed underscores in the right places, as 1.25 by default only supports AT&T syntax. I guess the best way to illustrate it is to compile the compiler twice, once as the AT&T compiler, and the next being the BSD compiler, and compare their output:
cc1-att.exe hi.cpp -quiet -dumpbase hi.c -version -o hi-att.s
GNU C version 1.25 (80386, ATT syntax) compiled by GNU C version 4.8.1.
.text
.LC0:
.byte 0x31,0x2e,0x32,0x35,0x0
.LC1:
.byte 0x68,0x65,0x6c,0x6c,0x6f,0x20,0x66,0x72,0x6f,0x6d
.byte 0x20,0x25,0x73,0xa,0x0
.align 2
.globl main
main:
pushl %ebp
movl %esp,%ebp
pushl $.LC0
pushl $.LC1
call printf
.L1:
leave
ret
And then looking at the BSD syntax:
cc1-bsd.exe hi.cpp -quiet -dumpbase hi.c -version -o hi-bsd.s
GNU C version 1.25 (80386, BSD syntax) compiled by GNU C version 4.8.1.
.file "hi.c"
.text
LC0:
.byte 0x31,0x2e,0x32,0x35,0x0
LC1:
.byte 0x68,0x65,0x6c,0x6c,0x6f,0x20,0x66,0x72,0x6f,0x6d
.byte 0x20,0x25,0x73,0xa,0x0
.align 1
.globl _main
_main:
pushl %ebp
movl %esp,%ebp
pushl $LC0
pushl $LC1
call _printf
L1:
leave
ret
As you can see main: becomes _main:, just as labels (LC0/LC1) have a prepended, while in BSD they do not. There are no doubt countless other nuanced differences, but for the assembler & operating system to match you want these to align. Thankfully calling conventions are mostly the same per processor so you can add the underscores to the AT&T target and get something that’ll run, not only on DJGPP but also Win32, as MinGW32 uses BSD syntax at its heart!
C:\xdjgpp.v1\src>gcc hi-bsd.s -o hi.exe
C:\xdjgpp.v1\src>wsl file hi.exe
hi.exe: PE32 executable (console) Intel 80386, for MS Windows
C:\xdjgpp.v1\src>hi
hello from 1.25
Not that we really need to go all the way and have GCC 1.25 running on anything much, although at the same time it’s kind of fun!
Reaching out for help
The oldest & most robust GCC is 1.27, and I’d been able to use that to build DooM before, but the caveat is of course the fixed point math. I’d asked smarter people than I years ago about this problem, and basically was told to figure it out for myself. After all its ‘trivial’. But alas, I’m not smart. What I would do is build the fixed point math with GCC and try to re-work that into other compilers, although again for platforms without GCC or the target CPU lacking the 64bit data type is well.. fatal.
But AI, sadly for it, is compelled to help. So I just went ahead and asked and got a surprising result!
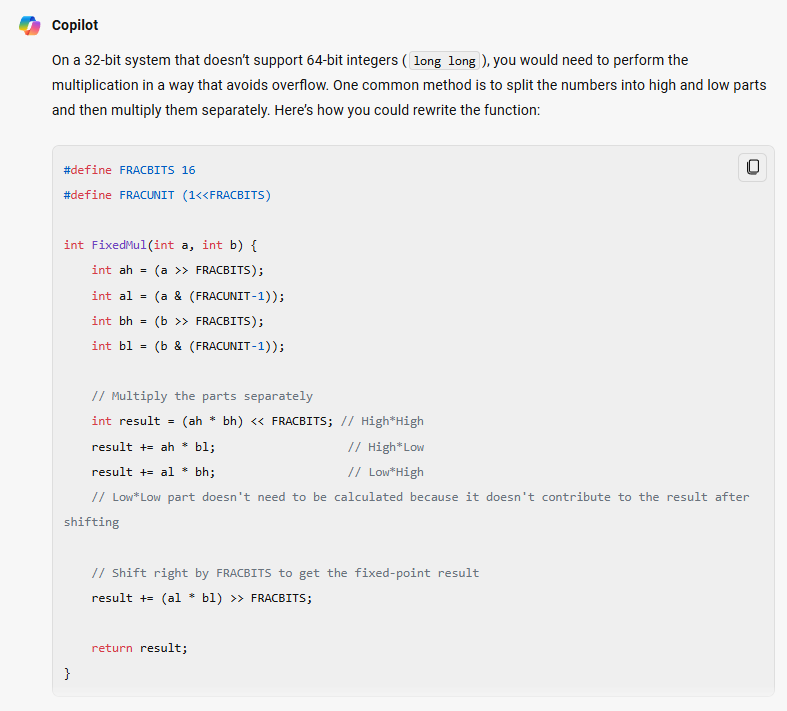
It’s very C++ like but it’s trivial enough to make it into old C.
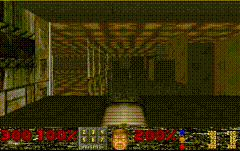
Well on the one hand it does actually load up and play. But the controls go wild and I’m pulled into the intersection of these boxes, and unable to move. And as I rotate the floor and walls clip in and out. It’s very weird.
Not knowing anything about anything, I saw this ‘guard’ on the fixed division and tried to add that to the multiply:
if ( (abs(a)>>14) >= abs(b))
return (a^b)<0 ? MININT : MAXINT;
And I got something even weirder!
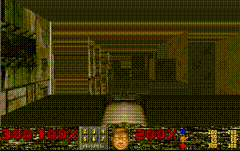
Not only that but the engine crashes! Not good.
After thinking about it on and off, asking for more help and going nowhere, I just gave up. It’s something beyond my skill, and apparently the AI as well. Until I had one of those moments in a dream where I had somehow told myself I bet the integers are not unsigned, and obviously fixed-point math needs all the bits, and it’s such a trivial fix, that even I should have figured it out.
I woke up at 4am and fired up the computer to see if it did anything. I was surprised to see that yes, in fact the integers were signed. I added the one key word, and recompiled using GCC 2.2:
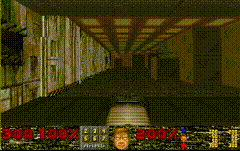
And it RAN! I tried GCC 1.39, and too ran! I then made sure there was no assembly modules being called accidentally, and then re-built with GCC 1.27, and yeah, it runs!
Armed with a simple port of DooM to Win32, I went ahead and put in the fixed-point solution, and used Visual C++ 1.10 aka Microsoft C/C++ 8.0 to build DooM, and yes it works there too!
In the end I guarded it around a long long type, as I’m sure it’s much more faster, but for those without the types or any assembly skill, here is the solution:
/* Fixme. __USE_C_FIXED__ or something. */
fixed_t
FixedMul
( fixed_t a,
fixed_t b )
{
#ifdef HAVE_LONG_LONG
return ((long long) a * (long long) b) >> FRACBITS;
#else
unsigned int ah,al,bh,bl,result;
ah = (a >> FRACBITS);
al = (a & (FRACUNIT-1));
bh = (b >> FRACBITS);
bl = (b & (FRACUNIT-1));
/* Multiply the parts separately */
result = (ah * bh) << FRACBITS; /* High*High */
result += ah * bl; /* High*Low */
result += al * bh; /* Low*High */
/* Low*Low part doesn't need to be calculated because it doesn't contribute to the result after shifting
// Shift right by FRACBITS to get the fixed-point result */
result += (al * bl) >> FRACBITS;
return (fixed_t)result;
#endif
}
/* */
/* FixedDiv, C version. */
/* */
fixed_t
FixedDiv2
( fixed_t a,
fixed_t b )
{
#ifdef HAVE_LONG_LONG
long long c;
c = ((long long)a<<16) / ((long long)b);
return (fixed_t) c;
#else
double c;
c = ((double)a) / ((double)b) * FRACUNIT;
if (c >= 2147483648.0 || c < -2147483648.0)
I_Error("FixedDiv: divide by zero");
return (fixed_t) c;
#endif
}
fixed_t
FixedDiv
( fixed_t a,
fixed_t b )
{
if ( (abs(a)>>14) >= abs(b))
return (a^b)<0 ? MININT : MAXINT;
return FixedDiv2 (a,b);
}
I haven’t tested it on Big Endian machines yet. I’ve updated my terrible DooM engine port, along with Doom-New for DOS. Additionally, I’ve been able to confirm it works with Watcom 9-OpenWatcom 2. It might even work with 7 & 8 but I haven’t tried.